import matplotlib.pyplot as plt
import numpy as np
def plot_bar_chart(data, labels, colors=None, title="Bar Chart", xlabel="X-Axis", ylabel="Y-Axis"):
"""
绘制柱状图,并在柱子上显示数量和比例。
:param data: 包含数值的列表。
:param labels: 与数据相对应的标签列表。
:param colors: 柱子的颜色列表。
:param title: 图表的标题。
:param xlabel: X轴的标签。
:param ylabel: Y轴的标签。
"""
fig, ax = plt.subplots()
bars = plt.bar(labels, data, color=colors)
total = sum(data)
for bar in bars:
yval = bar.get_height()
plt.text(bar.get_x() + bar.get_width()/2, yval, f'{yval}\n({yval/total:.1%})', ha='center', va='bottom')
plt.title(title)
plt.xlabel(xlabel)
plt.ylabel(ylabel)
plt.show()
# 示例数据
simple_data = [15, 30, 45, 10]
simple_labels = ['A', 'B', 'C', 'D']
simple_colors = ['red', 'green', 'blue', 'orange']
grouped_data = [[12, 30, 1, 8], [28, 6, 16, 5]]
group_labels = ['Group 1', 'Group 2', 'Group 3', 'Group 4']
category_labels = ['Category A', 'Category B']
# 绘制图表
plot_bar_chart(simple_data, simple_labels, simple_colors, title="Simple Bar Chart", xlabel="Category",ylabel="Values")
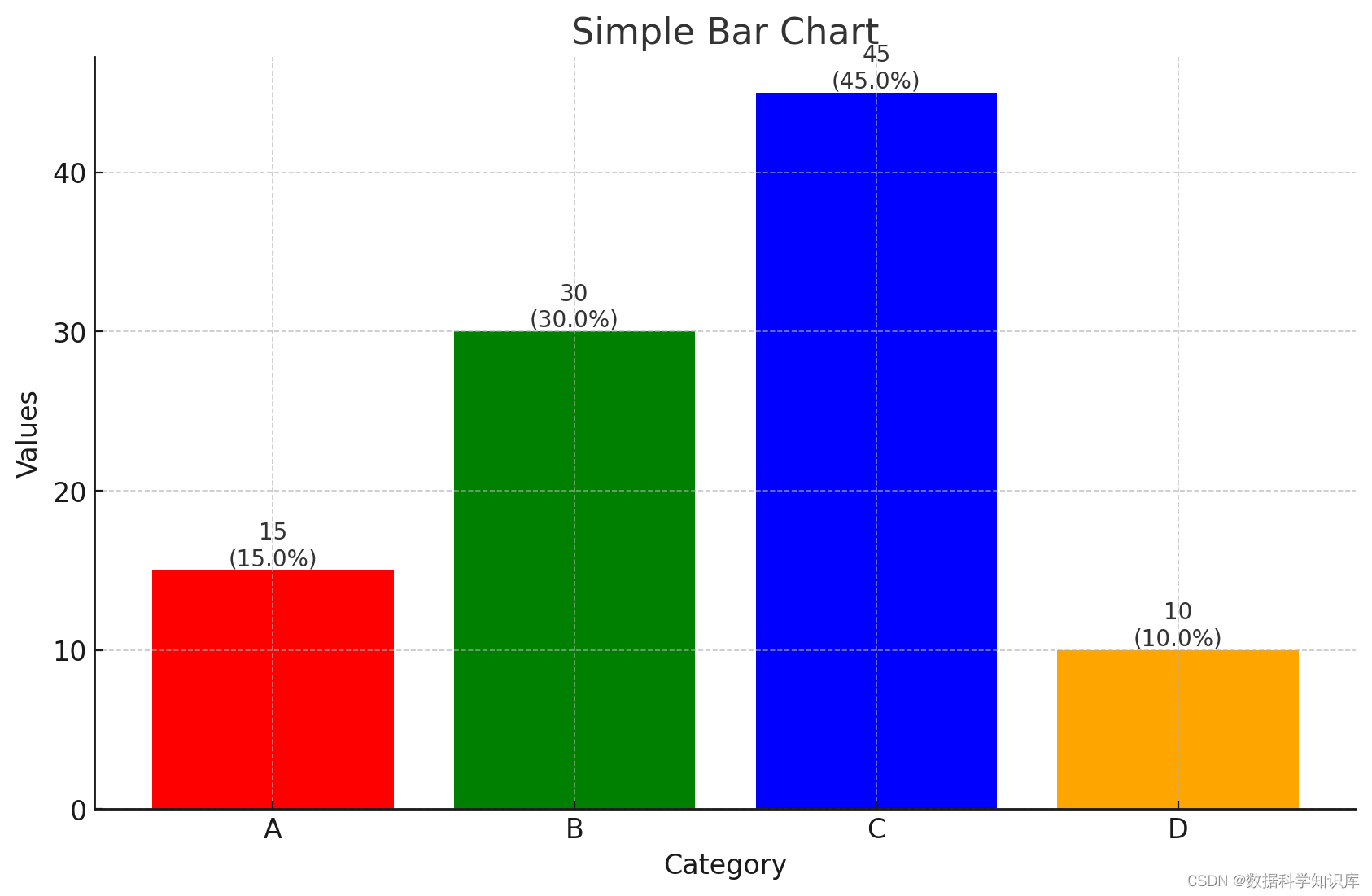
import matplotlib.pyplot as plt
import numpy as np
def plot_grouped_bar_chart(data, group_labels, category_labels, colors=None, title="Grouped Bar Chart", xlabel="X-Axis", ylabel="Y-Axis"):
"""
绘制分组柱状图,并在柱子上显示数量和比例。
:param data: 一个二维列表,其中每个内部列表包含同一组的数据。
:param group_labels: 每组的标签列表。
:param category_labels: 类别标签列表。
:param colors: 每个类别的柱子颜色列表。
:param title: 图表的标题。
:param xlabel: X轴的标签。
:param ylabel: Y轴的标签。
"""
n_groups = len(data[0])
n_categories = len(data)
fig, ax = plt.subplots()
index = np.arange(n_groups)
bar_width = 0.35
opacity = 0.8
if not colors or len(colors) != n_categories:
colors = plt.cm.viridis(np.linspace(0, 1, n_categories))
total = np.sum(data, axis=0)
for i in range(n_categories):
bars = plt.bar(index + i * bar_width, data[i], bar_width, alpha=opacity, color=colors[i], label=category_labels[i])
for bar, total_val in zip(bars, total):
yval = bar.get_height()
plt.text(bar.get_x() + bar.get_width()/2, yval, f'{yval/total_val:.1%}', ha='center', va='bottom')
plt.title(title)
plt.xlabel(xlabel)
plt.ylabel(ylabel)
plt.xticks(index + bar_width / 2, group_labels)
plt.legend()
plt.tight_layout()
plt.show()
# 示例数据
grouped_data = [[12, 30, 1, 8], [28, 6, 16, 5]]
group_labels = ['Group 1', 'Group 2', 'Group 3', 'Group 4']
category_labels = ['Category A', 'Category B']
# 绘制图表
plot_grouped_bar_chart(grouped_data, group_labels, category_labels, title="Grouped Bar Chart", xlabel="Groups",ylabel="Values")
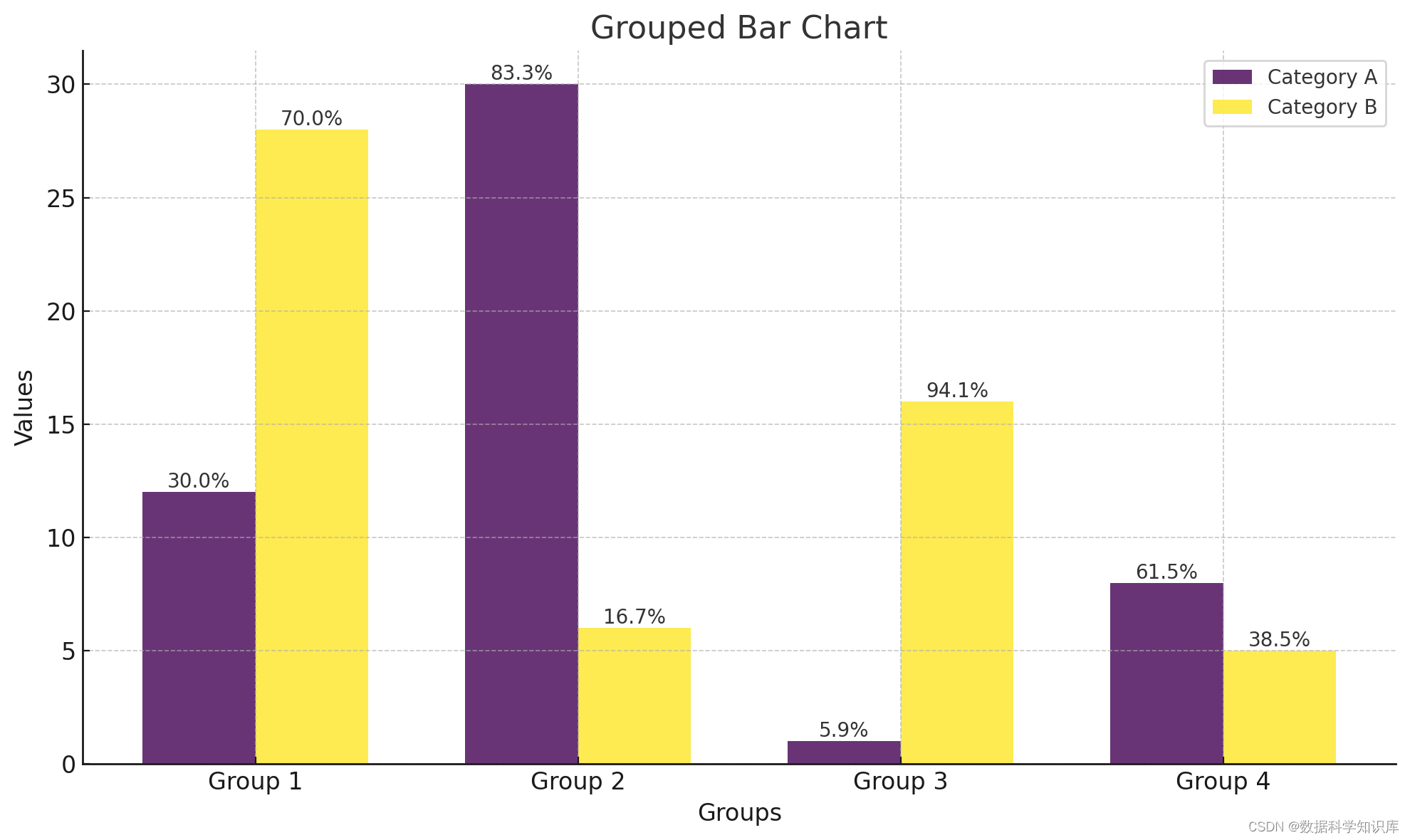